Convert camera images to pixels on a s square grid¶
[1]:
from ctapipe.utils import get_dataset_path
from ctapipe.visualization import CameraDisplay
from ctapipe.instrument import SubarrayDescription
from ctapipe.io import EventSource
from ctapipe.image.toymodel import Gaussian
import matplotlib.pyplot as plt
import astropy.units as u
[2]:
# get the subarray from an example file
subarray = SubarrayDescription.read("dataset://gamma_prod5.simtel.zst")
Geometries with square pixels¶
Define a camera geometry and generate a dummy image:
[3]:
geom = subarray.tel[40].camera.geometry
model = Gaussian(
x=0.05 * u.m,
y=0.05 * u.m,
width=0.01 * u.m,
length=0.05 * u.m,
psi="30d",
)
_, image, _ = model.generate_image(geom, intensity=500, nsb_level_pe=3)
[4]:
CameraDisplay(geom, image)
[4]:
<ctapipe.visualization.mpl_camera.CameraDisplay at 0x7f962e5a8670>
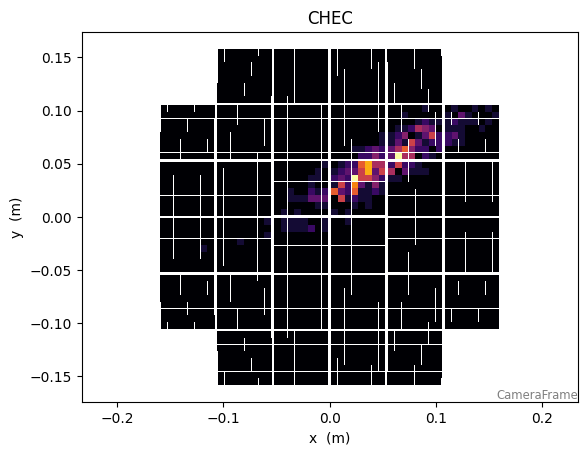
The CameraGeometry
has functions to convert the 1d image arrays to 2d arrays and back to the 1d array:
[5]:
image_square = geom.image_to_cartesian_representation(image)
[6]:
plt.imshow(image_square)
[6]:
<matplotlib.image.AxesImage at 0x7f962df0c3d0>
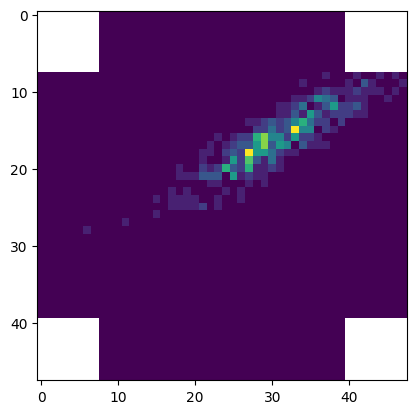
[7]:
image_1d = geom.image_from_cartesian_representation(image_square)
[8]:
CameraDisplay(geom, image_1d)
[8]:
<ctapipe.visualization.mpl_camera.CameraDisplay at 0x7f962c84b0a0>
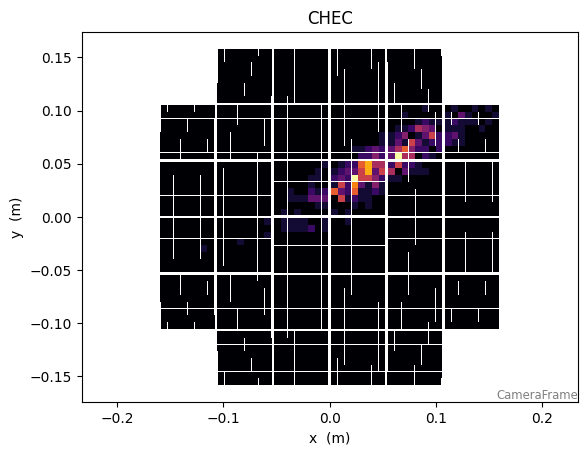
Geometries with hexagonal pixels¶
Define a camera geometry and generate a dummy image:
[9]:
geom = subarray.tel[1].camera.geometry
model = Gaussian(
x=0.5 * u.m,
y=0.5 * u.m,
width=0.1 * u.m,
length=0.2 * u.m,
psi="30d",
)
_, image, _ = model.generate_image(geom, intensity=5000)
[10]:
CameraDisplay(geom, image)
[10]:
<ctapipe.visualization.mpl_camera.CameraDisplay at 0x7f962e5b7e80>
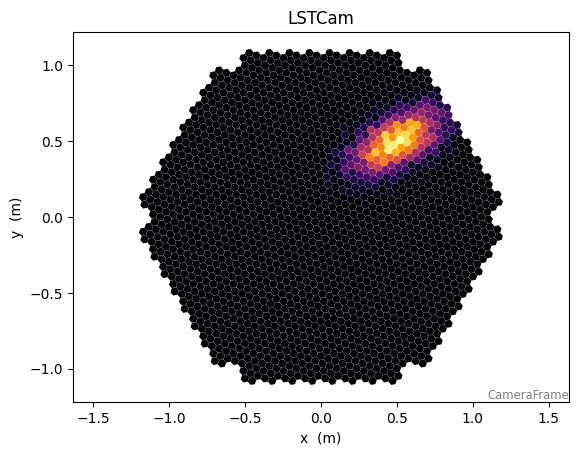
[11]:
image_square = geom.image_to_cartesian_representation(image)
Conversion into square geometry¶
Since the resulting array has square pixels, the pixel grid has to be rotated and distorted. This is reversible (The image_from_cartesian_representation
method takes care of this):
[12]:
plt.imshow(image_square)
[12]:
<matplotlib.image.AxesImage at 0x7f962ebf96d0>
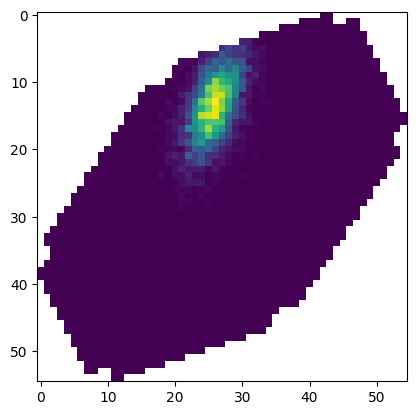
[13]:
image_1d = geom.image_from_cartesian_representation(image_square)
[14]:
disp = CameraDisplay(geom, image_1d)
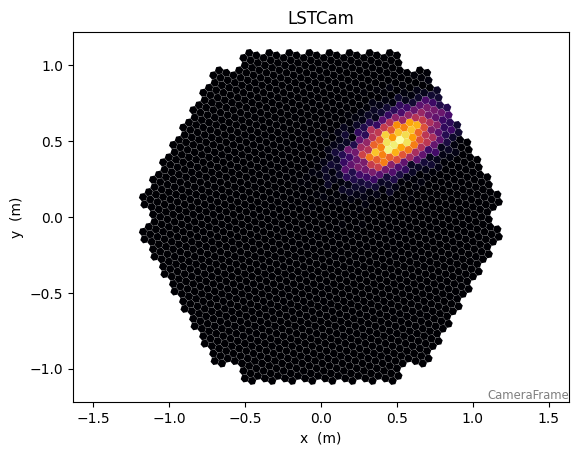