Camera Geometries¶
The CameraGeometry
provides an easy way to work with images or data
cubes related to Cherenkov Cameras. In ctapipe, a camera image is
simply a flat 1D array (or 2D if time information is included), where
there is one value per pixel. Of course, to work with such an array,
one needs spatial information about how the pixels are laid out.
Since CTA has at least 6 different camera types, and may have multiple
versions of each as revisions are made, it is necessary to have a
common way to describe all cameras.
So far there are several ways to construct a CameraGeometry
:
EventSource
instances have asubarray
attribute, e.g. to obtain the geometry for the telescope with id 1, use:source.subarray.tel[1].camera.geometry
.TableLoader
also has the.subarray
attribute.use the
CameraGeometry
constructor, where one has to specify all necessary information (pixel positions, types, areas, etc)load it from a pre-written file (which can be in any format supported by
astropy.table
, as long as that format allows for header-keywords as well as table entries.
Once loaded, the CameraGeometry
object gives you access the pixel
positions, areas, neighbors, and shapes.
CameraGeometry
is used by most image processing algorithms in the
ctapipe.image
module, as well as displays in the
ctapipe.visualization
module.
Input/Output¶
- You can write out a
CameraGeometry
by using theCameraGeometry.to_table()
- method to turn it into an
astropy.table.Table
, and then call itswrite
function. Reading it back in can be done with
CameraGeometry.from_table()
- method to turn it into an
geom = CameraGeometry(...) # constructed elsewhere
geom.to_table().write('mycam.fits.gz') # FITS output
geom.to_table().write('mycam.h5', path='/cameras/mycam') # hdf5 output
geom.to_table().write('mycam.ecsv', format='ascii.ecsv') # text table
# later read back in:
geom = CameraGeometry.from_table('mycam.ecsv', format='ascii.ecsv')
geom = CameraGeometry.from_table('mycam.fits.gz')
geom = CameraGeometry.from_table('mycam.h5', path='/cameras/mycam')
A note on Pixel Neighbors¶
The CameraGeometry
object provides two pixel-neighbor
representations: a neighbor adjacency list (in the neighbors
attribute) and a pixel adjacency matrix (in the neighbor_matrix
attribute). The former is a list of lists, where element i is a
list of neighbors j of the i*th pixel. The latter is a 2D matrix
where row *i is a boolean mask of pixels that are neighbors. It is
not necessary to load or specify either of these neighbor
representations when constructing a CameraGeometry
, since they
will be computed on-the-fly if left blank, using a KD-tree
nearest-neighbor algorithm.
It is recommended that all algorithms that need to be computationally
fast use the neighbor_matrix
attribute, particularly in conjunction
with numpy
operations, since it is quite speed-efficient.
Examples¶
from matplotlib import pyplot as plt
from ctapipe.instrument import SubarrayDescription
subarray = SubarrayDescription.read("dataset://gamma_prod5.simtel.zst")
geom = subarray.tel[1].camera.geometry
plt.figure(figsize=(8, 3))
plt.subplot(1, 2, 1)
plt.imshow(geom.neighbor_matrix, origin="lower")
plt.title("Pixel Neighbor Matrix")
plt.subplot(1, 2, 2)
plt.scatter(geom.pix_x, geom.pix_y)
plt.title("Pixel Positions")
(Source code
, png
, hires.png
, pdf
)
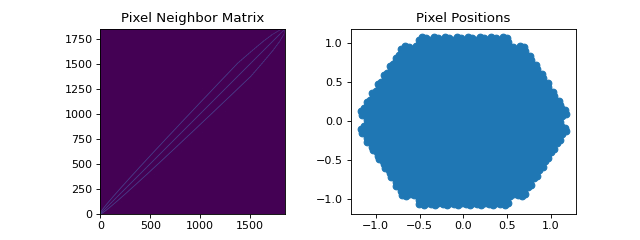
See also ctapipe.image.tailcuts_clean()
and ctapipe.image.dilate()
for usage examples.
Reference/API¶
ctapipe.instrument.camera.geometry Module¶
Utilities for reading or working with Camera geometry files
Classes¶
|
|
|
Supported Pixel Shapes Enum |